Haskell is a purely functional programming language that emphasizes immutability, mathematical rigor, and lazy evaluation. Since its inception, it has been a favorite among academic researchers and those who require high reliability and correctness in their code. Unlike imperative languages, Haskell relies on a declarative approach to programming, where you describe what the program should do, rather than how it should do it.
The History of Haskell
Haskell was born out of a need for a standardized functional programming language. In the 1980s, numerous functional programming languages were being developed, but each had its own unique syntax and semantics, making it difficult for researchers and educators to share their work. To address this, in 1987, a group of prominent computer scientists convened to create a standardized language. They aimed to develop a language that combined the best features of existing functional languages while being simple, clean, and suitable for teaching.
The first version of Haskell was released in 1990, named after the logician Haskell Curry, who made significant contributions to the field of combinatory logic and functional programming. Over the years, Haskell has evolved, with its most popular version, Haskell 98, being a widely accepted stable version used by both academia and industry. The latest versions continue to add new features while maintaining backward compatibility.
Key Features of Haskell
Haskell is distinct from other programming languages due to several key features:
- Pure Functions: In Haskell, functions are pure, meaning they always produce the same output for a given input and have no side effects. This makes the code more predictable and easier to test.
- Lazy Evaluation: Haskell does not evaluate expressions until their values are actually needed. This allows for powerful optimizations and the creation of infinite data structures.
- Immutability: Once a value is created, it cannot be modified. This helps prevent side effects and makes it easier to reason about the state of a program.
- Strong Static Typing: Haskell’s type system ensures that many errors are caught at compile time, reducing bugs and increasing the reliability of programs.
- Concurrency: Haskell has excellent support for concurrent and parallel programming, making it suitable for high-performance computing tasks.
Some Simple Examples of Haskell Code
To give you an idea of how Haskell works, let’s look at some simple examples.
Hello World in Haskell:
main :: IO ()
main = putStrLn "Hello, World!"
Add Two Numbers in Haskell:
add :: Int -> Int -> Int
add x y = x + y
Suppose we want to write a program to calculate the factorial of a number.
Authors Note ✏️
The factorial of a number is the product of all positive integers less than or equal to that number. It is denoted by the symbol n!
, where n
is the number. The factorial is defined as:
n! = n×(n−1)×(n−2)×⋯×3×2×1n! = n \times (n – 1) \times (n – 2) \times \dots \times 3 \times 2 \times 1n!=n×(n−1)×(n−2)×⋯×3×2×1
For example:
4!=4×3×2×1=244! = 4 \times 3 \times 2 \times 1 = 244!=4×3×2×1=24
5!=5×4×3×2×1=1205! = 5 \times 4 \times 3 \times 2 \times 1 = 1205!=5×4×3×2×1=120
Factorial in Haskell:
-- Factorial function using recursion
factorial :: Integer -> Integer
factorial 0 = 1
factorial n = n * factorial (n - 1)
Let’s break it down:
- The
factorial
function takes an integern
and returns its factorial. - The line
factorial 0 = 1
is the base case: the factorial of 0 is defined as 1. - The second line
factorial n = n * factorial (n - 1)
is the recursive case: it calls the function onn - 1
and multiplies the result byn
. - The type signature
factorial :: Integer -> Integer
indicates that the function takes anInteger
as input and returns anInteger
.
In Haskell, recursion is commonly used to define functions, as it allows for elegant and concise solutions to many problems.
Running the Code
To run the factorial function, you would simply call it in a Haskell environment (such as GHCi, the interactive Haskell interpreter):
factorial 5
-- Output: 120
This concise, recursive approach to calculating a factorial demonstrates the power and elegance of Haskell.
Haskell in the Real World
While Haskell’s origins are in academia, it has found a niche in the industry, particularly in areas that require strong correctness guarantees. Financial services companies, for example, use Haskell to write trading systems where reliability and correctness are paramount. Haskell is also used in the telecommunications industry and has gained traction in blockchain development, with the Cardano blockchain being one of the more prominent examples built using Haskell.
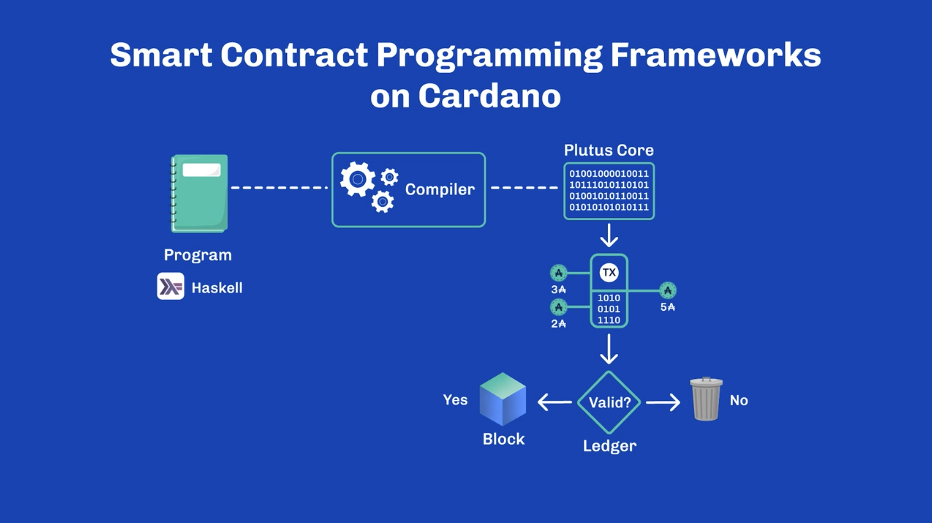
Benefits of Using Haskell
- Maintainable Code: Haskell’s strong typing and immutability make it easier to write maintainable, bug-free code.
- Concurrency and Parallelism: Haskell provides built-in support for concurrent and parallel programming, making it ideal for applications that need to scale efficiently.
- Mathematical Precision: Haskell is great for applications that require mathematical rigor and precision, such as financial systems.
Conclusion
Haskell is a language designed with a focus on purity, immutability, and mathematical correctness. Its roots in academia have shaped it into a language that prioritizes clean, maintainable code. While it can have a steep learning curve, its benefits, especially in areas like correctness and concurrency, make it a powerful tool for both researchers and industry professionals.
Whether you are interested in building highly reliable software systems or exploring the world of functional programming, Haskell is a language worth learning. Its unique approach to solving problems provides a fresh perspective on how we can design and write software.
💡 Helpful References
Learn You a Haskell for Great Good!
https://learnyouahaskell.github.io/introduction.html#about-this-tutorial
FAQs about Haskell
What is Haskell?
Haskell is a statically typed, purely functional programming language known for its strong type system, high-level abstractions, and lazy evaluation.
Why should I use Haskell?
Haskell is used for writing robust, maintainable, and efficient programs, especially in fields like financial modeling, blockchain development, and academic research. Its type system reduces runtime errors, and functional paradigms make reasoning about code easier.
What makes Haskell different from other programming languages?
Haskell is purely functional, meaning functions don’t have side effects. It also uses lazy evaluation, meaning expressions are not evaluated until they’re needed, improving efficiency in many scenarios.
Is Haskell difficult to learn?
Haskell can be challenging for beginners because of its unique paradigms like functional programming and type systems. However, once learned, it often provides significant benefits in writing concise, high-quality code.
What are some popular applications of Haskell?
Haskell is used in blockchain technology (e.g., Cardano), web development, compilers, and high-assurance systems like those in finance and aerospace.
How do I get started with Haskell?
You can start by installing the Haskell Platform, which includes the Glasgow Haskell Compiler (GHC), or using an online REPL. Haskell’s website and books like “Learn You a Haskell for Great Good!” are good learning resources.
What are monads in Haskell?
Monads are a design pattern used in Haskell to handle computations like state, I/O, and exceptions in a pure functional way. They allow chaining operations while managing side effects.
Is learning Haskell necessary for understanding Cardano?
Learning Haskell is not strictly necessary for using Cardano, but it can be highly beneficial depending on your goals:
If you’re a Cardano user or ADA holder:
- No, you don’t need to learn Haskell. You can use wallets like Daedalus or Yoroi for staking, sending, or receiving ADA without any programming knowledge.
If you’re a developer building on Cardano:
- It depends on the level of involvement. While it’s not mandatory for all developers, learning Haskell is helpful in specific areas:
- Plutus Smart Contracts: Plutus, the smart contract platform on Cardano, is based on Haskell. Learning Haskell will allow you to write and understand Plutus smart contracts effectively.
- Marlowe: If you’re working with Marlowe (Cardano’s DSL for financial contracts), it’s designed to be more accessible, and you won’t necessarily need Haskell. But having a background in Haskell can help understand the underlying concepts.
If you’re interested in Cardano’s core infrastructure:
- Yes, Haskell would be essential. The core components of Cardano, such as its consensus algorithm (Ouroboros), are written in Haskell. If you want to contribute to Cardano’s infrastructure or protocol development, you’ll need a solid understanding of Haskell.
In summary:
- For general use and basic development: Haskell is not necessary.
- For smart contract development and deeper protocol work: Learning Haskell is advantageous.
Leave a Reply